Introduction
Robot programming involves writing instructions for robots that enable them to autonomously complete tasks. Programming robots can be done in many different languages, but Python has become an increasingly popular choice due to its flexibility and ease of use. In this article, we will explore the basics of robot programming with Python and discuss the advantages and disadvantages of using it.
Basic Steps of Programming a Robot in Python
Programming a robot with Python can be divided into three main steps: setting up the environment, writing the code, and testing the code.
Setting up the Environment
The first step is to set up the programming environment. This includes installing the necessary software and connecting the robot to the computer. Depending on the type of robot you are using, there may also be other hardware requirements such as sensors or cameras. Once all the necessary components are in place, you can begin programming the robot.
Writing the Code
The second step is to write the code that will control the robot. The code should include instructions on how to move, sense the environment, and interact with other robots or objects. It should also include any necessary safety protocols to ensure the robot does not cause harm to itself or anyone else.
Testing the Code
The final step is to test the code. This can be done by running the code on the robot and observing its behavior. If any errors are found, they can be fixed before the robot is put into action.
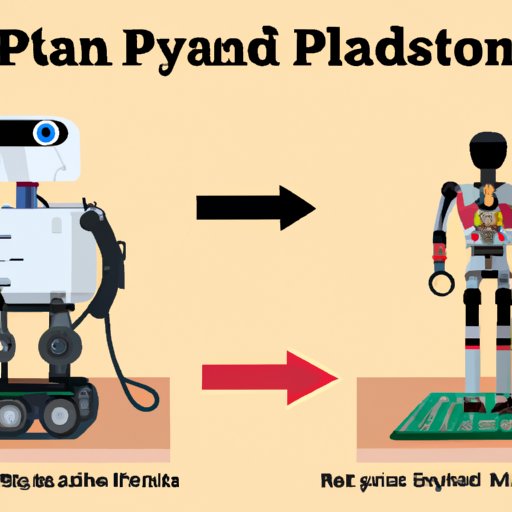
Advantages and Disadvantages of Using Python to Program Robots
There are several advantages and disadvantages to using Python to program robots. Let’s take a look at some of the most notable ones.
Advantages
Python is a versatile language that is easy to learn and understand. It is also open source, meaning it is free to use and modify. Furthermore, Python is widely used in robotics research, so there is a wealth of resources available to help new programmers get started. Finally, Python can be used to control both physical and virtual robots, making it a great choice for both real-world and simulated applications. As noted by Dr. Sean Luke, a professor at George Mason University, “Python is an excellent choice for robot programming due to its flexibility and straightforward syntax.”
Disadvantages
One potential disadvantage of using Python to program robots is its relatively slow execution speed. This can be a problem for complex tasks that require fast response times. Additionally, Python is not well suited for low-level tasks such as controlling motors or sensors. For these types of tasks, other languages like C++ may be more suitable.
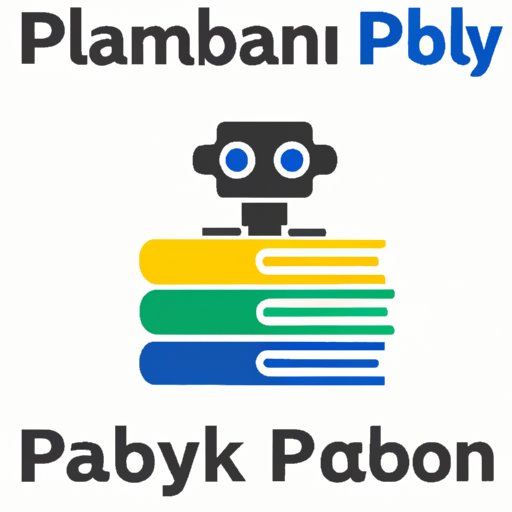
Libraries Available for Programming Robots with Python
There are a variety of libraries available for programming robots with Python. These libraries provide useful functions and classes that make it easier to program robots. Let’s take a look at some of the most popular libraries.
Overview of Libraries
The most popular libraries for programming robots with Python are ROS (Robot Operating System) and OpenCV (Open Source Computer Vision Library). ROS is a powerful open source framework for developing robotic applications. It includes a wide range of packages for working with sensors, actuators, and other robotic components. OpenCV is a library for working with images and videos. It can be used for facial recognition, object tracking, and other computer vision tasks.
Discussing Some Popular Libraries
ROS is the most popular library for programming robots with Python. It is designed to simplify the development process and provide a robust platform for building advanced robotic applications. Additionally, ROS provides a wide range of packages for working with various sensors and actuators. OpenCV is another popular library for robot programming. It can be used for image processing and computer vision tasks, such as facial recognition and object tracking. Other popular libraries include PyGame and TensorFlow, which can be used for machine learning applications.
Writing Code to Control a Robot’s Movements
Once the necessary libraries are installed, the next step is to write code to control the robot’s movements. This can be done by calling functions from the libraries and passing in parameters to control the robot’s speed, direction, and other properties.
Overview of Functions
Most libraries provide functions for controlling the robot’s movements. For example, ROS provides the move_base() function for controlling a robot’s linear and angular velocities. Similarly, OpenCV provides the cv2.circle() function for drawing circles on an image.
Examples of Codes
Below are some examples of code for controlling a robot’s movements. The first example is a function for moving a robot forward 10 meters using the ROS move_base() function. The second example is a function for drawing a circle on an image using the OpenCV cv2.circle() function.
ROS example:
def move_forward(distance):
# Set linear velocity
linear_velocity = 0.1
# Set angular velocity
angular_velocity = 0.0
# Call move_base() function
move_base(linear_velocity, angular_velocity, distance)
OpenCV example:
def draw_circle(image, center, radius, color):
# Call cv2.circle() function
cv2.circle(image, center, radius, color, -1)
Showcase Examples of Robotic Projects Programmed with Python
Now that we have a better understanding of how to program robots with Python, let’s take a look at some showcase examples of robotic projects programmed with Python.
Introducing Different Projects
One example is a robot arm programmed with Python to pick and place objects placed on a conveyor belt. Another example is a self-driving car programmed with Python to navigate a given route. Finally, there is a robot programmed with Python to play soccer against other robots.
Explaining How They Were Programmed
The robot arm was programmed using ROS and OpenCV. The robot was trained to recognize different objects on the conveyor belt and then use its arm to pick them up and place them in designated locations. The self-driving car was programmed using TensorFlow and OpenCV. The robot was trained to identify and follow roads and lanes, as well as detect and avoid obstacles. Finally, the soccer-playing robot was programmed using ROS and OpenCV. The robot was trained to recognize the ball and other robots, and then use its motors to move around and kick the ball.

Troubleshooting Common Errors in Robot Programming with Python
Finally, let’s discuss some tips for troubleshooting common errors in robot programming with Python.
Best Practices for Debugging
When debugging a robot program, it is important to take a systematic approach. One approach is to break down the code into smaller pieces and test each one individually. This makes it easier to identify where the error is located. Additionally, it is important to keep track of the changes made to the code, as this can help identify what caused the error.
Tips for Identifying Errors
Another way to identify errors is to read through the error messages produced by the compiler or interpreter. These messages often provide clues about the source of the error. Additionally, it is helpful to print out intermediate values during the execution of the code, as this can help pinpoint where the problem is occurring. Finally, it is important to test the code frequently and make sure all the expected results are produced.
Conclusion
In conclusion, programming robots with Python is a great way to create autonomous applications. Python is a versatile language that is easy to learn and understand, and there are a variety of libraries available for robot programming. Additionally, there are several best practices for debugging and troubleshooting errors. With the right tools and techniques, anyone can start programming robots with Python.
(Note: Is this article not meeting your expectations? Do you have knowledge or insights to share? Unlock new opportunities and expand your reach by joining our authors team. Click Registration to join us and share your expertise with our readers.)