Introduction
Data structures are an essential part of computer science, as they provide a way to store and organize information. One type of data structure is called a stack. In this article, we will explore what a stack is, how it works, and its various practical applications in computer science.
A Step-by-Step Guide to Understanding Stack Data Structures
This guide will take you through the basics of stack data structures, from definition and structure to operations and practical uses. We’ll also look at some diagrams and examples to help you visualize how stacks work.
What is a Stack?
A stack is a linear data structure that stores items in a particular order. Items can be added or removed from the top of the stack, with the last item added being the first one removed. This process is known as Last-In, First-Out (LIFO).
How Does it Work?
A stack operates using two basic operations: push and pop. Push adds an item to the top of the stack, while pop removes the item at the top of the stack. These two operations allow for the efficient storage and retrieval of data.
Types of Stacks
There are several different types of stacks, including linked lists, arrays, and trees. Each type has its own advantages and disadvantages, so it’s important to consider which type of stack best meets your needs when designing a data structure.
Exploring the Basics: What is a Stack and How Does it Work?
Now that we have a general understanding of what a stack is and how it works, let’s dive deeper into its components.
Definition of a Stack
A stack is a collection of items that are stored and accessed in a particular order. The order of the items is determined by the push and pop operations. Push adds an item to the top of the stack, while pop removes the item at the top of the stack.
Structure of a Stack
A stack follows a Last-In, First-Out (LIFO) principle. This means that the last item pushed onto the stack is the first item popped off the stack. This allows for efficient storage and retrieval of data.
An Overview of Stacks: How Do They Function?
The operations of push and pop are used to access the items in a stack. Push adds an item to the top of the stack, while pop removes the item at the top of the stack. This allows for efficient storage and retrieval of data.
Push and Pop Operations
Push and pop are the two main operations used to access the items in a stack. Push adds an item to the top of the stack, while pop removes the item at the top of the stack. Both operations are necessary for efficient storage and retrieval of data.
Last-In, First-Out (LIFO) Principle
The Last-In, First-Out (LIFO) principle is the key to how a stack functions. This principle states that the last item pushed onto the stack is the first item popped off the stack. This allows for efficient storage and retrieval of data.
Practical Uses for Stacks in Computer Science
Stacks are used in a variety of ways in computer science. They are used in memory management, as well as in recursive algorithms. They are also used in programming languages such as Java and C++.
Memory Management
Stacks are used in memory management to store data efficiently. When a program needs to use a certain amount of memory, it pushes the data onto the stack. When the program is done using the data, it pops the data off the stack.
Recursion
Stacks are also used in recursive algorithms. Recursive algorithms are algorithms that call themselves until a certain condition is met. Stacks are used to store the data associated with each call of the algorithm, allowing the program to efficiently keep track of the calls.
Visualizing Stacks: How do they Operate?
Now that we’ve explored the basics of stacks, let’s take a look at how they work visually. We’ll look at diagrams of stacks, as well as some examples of stacks in action.
Diagrams of Stacks
Diagrams can help us visualize how stacks work. A diagram of a stack shows the items stored in the stack, as well as the push and pop operations used to add and remove items. This helps us understand how stacks work and how they can be used to store and retrieve data.
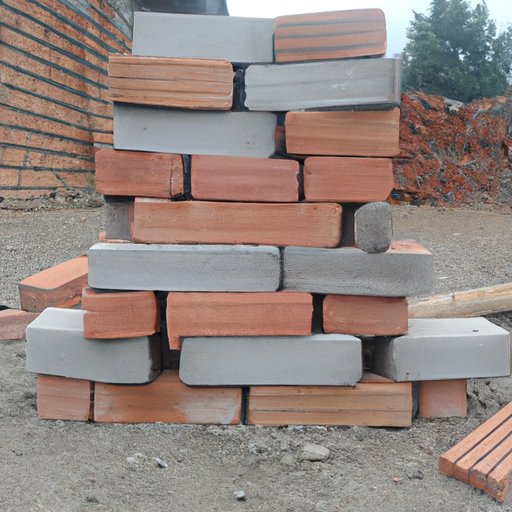
Examples of Stacks in Action
Examples of stacks in action can also help us understand how they work. For example, let’s say we have a stack of books. If we want to add a book to the stack, we would use the push operation to add it to the top of the stack. If we wanted to remove a book from the stack, we would use the pop operation to remove the book from the top of the stack.
Conclusion
In this article, we explored the basics of stack data structures. We looked at what a stack is, how it works, and its various practical applications in computer science. We also looked at diagrams and examples to help visualize how stacks work. Now that you have a better understanding of stack data structures, you can implement them in your own projects.
Summary of Key Points
Stack data structures are collections of items stored and accessed in a particular order. The two main operations used to access the items in a stack are push and pop. Stacks are used in memory management and recursive algorithms, as well as in programming languages such as Java and C++. Diagrams and examples can help us visualize how stacks work.
Resources for Further Learning
If you’d like to learn more about stack data structures, there are plenty of resources available online. Some good starting points include the following: “Data Structures and Algorithms in Java” by Robert Lafore, “Data Structures and Algorithms” by Michael T. Goodrich, and the Stack Overflow website.
(Note: Is this article not meeting your expectations? Do you have knowledge or insights to share? Unlock new opportunities and expand your reach by joining our authors team. Click Registration to join us and share your expertise with our readers.)